How to improve your JavaScript career
In this post, we are going to explore several topics that will have a meaningful impact on a professional programmer's career.
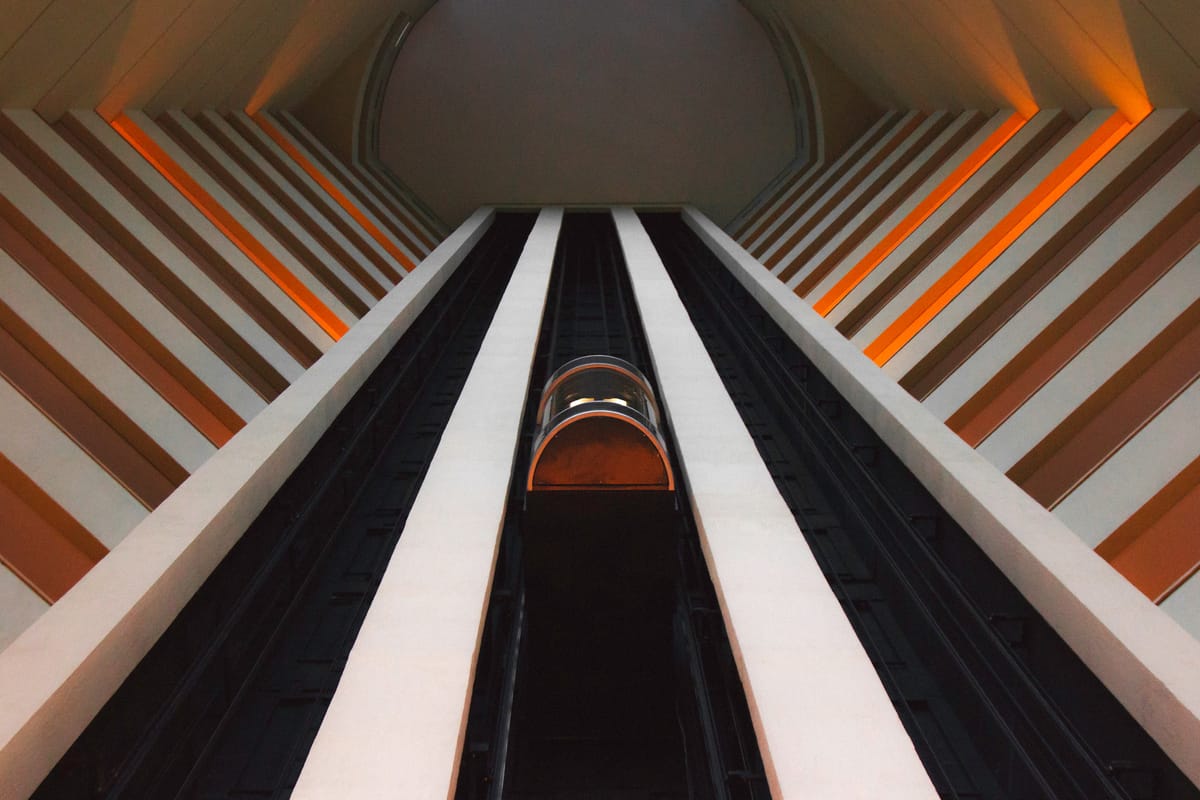
The goal of this post is to either present questions or provide a direction for learning. We may explore the individual topics presented here in future posts, but this is designed to guide a professionals research. Links have been provided to additional resources, it is recommended to take advantage of those as well.
Knowing how to program with JavaScript these days is a fantastic opportunity. Companies all over the country are scrambling to get more JavaScript programmers. Within the recent decade JavaScript has evolved from what some would consider a toy language, in part due to the lack of reasonable tooling and program management, into a programming language that is powerful and fun to write. JavaScript programmers are known as innovators, trendy, and chasers of innovation.
In this post, we are going to explore several topics that will have a meaningful impact on a professional programmer's career.
Master the basics
While there are plenty of tools, frameworks, and libraries to learn and enjoy, a powerful personal development technique, a programmer can practice is to master the fundamentals of their language. The fundamental principle to dedicate mastering the essential elements of a subject applies across many domains. By focusing on being a JavaScript programmer first, it sets one up to understand principals within frameworks and libraries. Select frameworks and libraries by their principals in combination with the features they provide. Mastering the basics in this way separates programmers that hammer tooling together and those that make choices with meaning.
Before diving into the next library, framework or interview question consider mastering these topics about JavaScript first.
Understand closures
Good JavaScript interviews will cover a candidates ability to reason about how closures work. Closures are critical to understand how to encapsulate and reveal information to a program or parts of a program. Being comfortable with closures a programmer will have the ability to strategically control the visibility of the parts of their program. Those that do not understand how a closure works pose a risk to a team, which is why many technical interviews cover closures directly.
- Understand when a closure is created for every function.
- Understand that inner closures have visibility into the closure that contains them, but not the other way around.
As a jumping off point, checkout MDN for a good explanation of closures. For an inspirational reference, why these constructs are named closures to begin with, look to the mathematical definition.
Understand context
The term "context" is the setting of an environment for an event. In interviews, programmers, including senior candidates, are sometimes surprised when asked to describe the characteristics of context. We have already explored this in closures, but understanding how to describe and control these features is relevant. In interviews expect to answer questions like:
- Describe the significance of
call
,apply
andbind
. - Understand the steps that occur when using
new
keyword with a function. For exampleconst john = new Person("John", "Smith")
. - Understand
this
. What is it for and how is it assigned?
Most top tech companies are asking these questions, and we stand to benefit as programmers to understand them. It is essential not to overlook context. Context is an integral part of the language that can have a lasting impact on how JavaScript programs are designed.
Explore context further as it relates to the keyword this
on MDN.
Understand lexical and block-level scope
Understanding what values or references are available where and controlling their visibility to other parts of a routine is excellent for keeping control over operations happening in an application. There has been a transition period in the JavaScript language for programmers to move from ECMAScript 5 to the 2016+ syntax.
- What is the difference between var, let and const?
- Describe when variable hoisting occurs.
- Describe the temporal dead zone and how it is similar and dissimilar to how variable hoisting works?
Understand the function and its characteristics
Functions can be created at anytime and passed around a JavaScript program and perform its role as the designer (or client) sees fit. Functions have several essential features. Take a look at the questions and statements below for an idea on what to study about the function in JavaScript:
- How to control the context of a function.
- What are the differences between named and anonymous functions?
- Implement currying at least one time and think about how to work with arguments. Where might a pattern like currying be helpful (Hint: Redux)?
- Write a JavaScript function that acts as a constructor, then append new fields to its prototype and inspect the results. (Hint:
console.dir({})
)
Acing the function is excellent for writing JavaScript. Functions carry the load as it applies to executing work, and this know-how is transferable into methods later on. While some terms may seem foreign they are relevant in modern design patterns like flux, functional and the observer pattern, where work is segregated into the smallest parts possible to later be composed together while maintaining as much purity as possible.
A worthwhile course to consider would be Chris Mather's short but deep course on the JavaScript function. While courses like these will cost some money, the quality is very high. Get to know what the term "first-class" means, all the way through an introduction of functional patterns.
Understand the characteristics of the Object
Objects are an essential building block for working with JavaScript. These key-value stores of paired bits of mixed types are powerful and a critical part of the programs we create with JavaScript. There are a number of details to consider for the object structure and the Object API.
- Understand the various ways objects may be created, including the Object API.
- Understand how are methods inherited from one object to another.
- Understand how to instantiate an object while excluding a prototype.
- Explain what writable, enumerable, or configurable means to someone.
- What is the significance of
Object.freeze()
? - Understand methods that provide introspection or validation on values an object may contain. For example, explore
hasOwnProperty
. Knowing that methods that are accessed by the prototype what would be a reasonable strategy for ensuring that the code execution is what was intended. For example, what if the object that was asked to performhasOwnProperty
was assigned a newhasOwnProperty
method? - Understand what objects OWNED property is and how it plays a role in the property access flow when accessing values on an object.
- Understand how to set or get dynamic fields on an object using bracket notation.
- Understand Garbage collection and how references to objects have an impact on the memory footprint of a program.
- Understand how to select all of the existing object's keys or values using methods like
Object.keys
orObject.values
.
Working into the strengths of objects and the object API will yield better programs through optimized memory usage and safety while providing more straightforward solutions.
Understand the underlying inheritance model
Know what the "own" space is in objects, understand references, and prototype chaining. Understanding prototypal inheritance may require taking a step back into the basics of computer science to learn more about how memory is allocated for a program and how pointers works, but this knowledge is transferable to other topics as well.
A thorough understanding of prototypal inheritance will clearly describe the following:
- Prototypal inheritance, done well, reduces the memory footprint of a JavaScript application.
- Understand what prototype fields are eligible for enumeration.
- That JavaScript classes are still entirely based on writing to the prototype and that the class structure is only sugar.
- Develop an opinion as to why Douglas Crockford considers the
prototype
in JavaScript a potential "bad part"? - What are the key arguments for disallowing classes from JavaScript?
- Practice overwriting methods inherited by the prototype chain. Overwriting methods in this way is commonly considered a bad practice, but there is nothing wrong with experimenting with what the language allows (mainly for debugging).
Enumeration, iteration, and relevant data structures
Programs commonly require the manipulation of data, and in many cases - a significant amount of data. While we explore objects, we understand key-value pairs. We learn how to store and organize information, but iteration and enumeration in many ways bring these concepts to life and make programs possible. Iteration techniques are also areas of programs; many of us will spend time to design means for optimization. Professional programmers and engineers will seek out places iteration occurs in applications and design strategies that mitigate the amount of computing (work) that is required to achieve the goal of a routine.
A quick note on data structures in JavaScript
JavaScript lacks API's for data structures like queues and stacks (at least as of this writing), but JavaScript has the makings of these elements baked into how arrays work.
- Understand big O notation. Big O notation provides us a language to describe the performance of algorithms. Time is the target measure here and O is seeking to understand the worst case scenario.
- Understand stack and queue data structures.
- Understand the different ways of creating arrays, for example, create an array with no elements, a collection with some items, and create a new array comprised of elements from the two other arrays.
- Understand the native array methods, such as
map
,reduce
,filter
, andsome
. Pay close attention to the return types; for instance, many folks do not realize that the result of some of these array methods returns a new array or value. - Understanding looping like the for, for each, for in and while loops.
- Understand how to break out of a loop early. Breaking out early from loops will prevent other work from happening, and less work means better performance.
- What type of data structure is the DOM? (Tip: this a common gateway interview question).
Understand asynchronous programming
When speaking about asynchronous programming with JavaScript, the one answer that always comes up in conversation is making requests to an external API. Chatter between a JavaScript application and an API is only the beginning of asynchronous programming. Asynchronous programming continues to grow in popularity in JavaScript, Java, and languages like Rust. JavaScript provides constructs to create programs that read in an imperative style but execute using asynchronous models. Imagine being able to allow a program to continue with other things while a complex computation is being worked on behind the scenes. Asynchronous techniques keep applications feeling responsive even while under heavy computational load.
- Know the difference between blocking and non-blocking workflows.
- Spend time with the fetch API.
- Read up on Promise and Promise.all.
- Understand async/await.
- Practice: loop to 500,000 in an nonblocking manner.
- Understand Promises and the A+ promise specification.
- Spend a little bit of time on the event loop in Node.js.
This list of asynchronous topics is not exhaustive, and these topics will be on the mind-bending side of things for those not already comfortable with designing programs that do not read like instruction manuals. Be patient while exploring asynchronous programming, asynchronous programming is compelling for JavaScript and will be a superpower for lower-level languages like Rust. Performant JavaScript applications leverage asynchronous programming in meaningful and strategic ways.
Practice Test (Design) Driven Development
The benefits of TDD are only felt through practice. Those of us that argue against TDD is typically not well-practiced, and those of us that say for it have felt its benefits. Practicing TDD is not enough. TDD is littered with mixed practices and struggles to make itself apparent as a discipline.
- Understand mocking.
- Learn the difference between an assertion language like Jasmine and a testing framework like Jest.
- Explore the phrase "Do not Mock What you Do not Own."
- Understand the spy.
- How to work with testing asynchronous code.
- What is a good test?
- Identify whether or not a test is covering what is expected.
For fun, take a look at mutation testing. Advanced teams consider the quality of their test suite by test accuracy, when and how the tests run.
Study programming design patterns
Patterns come from the continued practice of programming. In computer languages, patterns help humans organize their thoughts around different types of problems in similar ways. While working on medium to large size applications, the use of patterns will help a team stay consistent and their programs extendable.
One of the best, and free, bodies of work available on the topic of design patterns with JavaScript would be Learning JavaScript Design Patterns by Addy Osmani. Knowing patterns allows us to design computer language in common ways around any problem.
Finding the next step
Here are some thoughts on where to go next based on experience level:
Reading this is overwhelming
Those of us that may be confused, a better place to begin, will be in an entry-level programming lesson. A couple of great places to get started could be:
The topic of writing programs is complicated, but it is within the grasp of any consistent person's ability to learn.
How to learn more about X
There are many topics covered here, and we very well may explore then more deeply in future posts. However, do not be afraid to read the ECMAScript language specification. Learning from the language spec may take a few tries to understand, but with practice, things will make more sense. Some resources include:
About web development
Web development requires several additional topics that are worth exploring. For instance, the DOM and CSS. While these are disciplines onto themselves, they are essential to understand as separate topics.
This is missing a few things
Of course, some things were missed! Feedback may be provided in the comments on this platform or through email.