Meteor methods: How to provide a callback for error and success scenerios
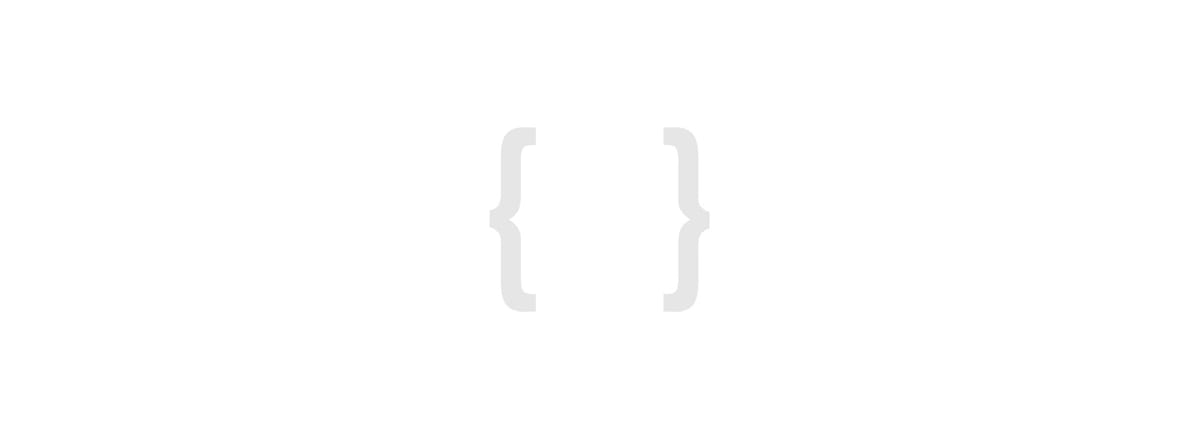
In this tutorial will make the following assumptions:
- That we have a document set we are acting upon that is provided the
Schedule
symbol to be acted against. This is common inMeteor.js
applications. - We are using ES2015+
- The code you are about to see is not optimized for production. Your methods should include
check
validation etc... This is for study case only.
TLDR
Return the collection modification from your meteor method.
Meteor.method({
'Method.name'( newDocument ) {
return Schedule.insert( newDocument );
}
});
This tutorial is to help you figure out how to handle the potential error or result arguments while developing Meteor
server methods that do some database transaction.
First, we need a Meteor
method to call that will require some update to a collection of ours.
Meteor.method(
'Schedule.add-new'( schedule ) {
Schedule.insert( schedule );
}
);
Now when we call Schedule.add-new
we typically expect to pass some callback function as the final argument to handle error, result
cases.
Meteor.call( 'Schedule.add-new', {
sectionTitle: 'New Section',
tasks: [],
}, ( error, result ) => {
if( error ) {
throw new Meteor.Error( error.message );
} else if( result ) {
console.log( result ); // document GUID
}
} );
Our usage of our Meteor method is fine, but what we will find is that we will always see undefined
being returned from our server method. The reason being is that our method is asyncronous, so the method will return before our call to insert Schedule
is ever completed.
We can fix this easily by returning a handle to our collection work.
Meteor.methods( {
'Schedule.add-new'( schedule ) {
return Schedule.insert( schedule );
}
} );
Now your callback will be provided with the expected error
and result
arguments.